An I2C keypad for the micro:bit - part 2
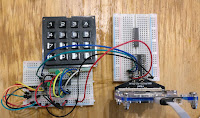
A working prototype I've now got a home-brew Arduino clone reading the keyboard and sending key-presses to a micro:bit using I2C. Preparing the Arduino clone was trickier than usual. As I mentioned in yesterday's post , the Arduino needs to run at 3.3 volts. That's the working voltage (V cc ) for the micro:bit, the Pi and the Jetson Nano. Connecting I2C lines from a 5v device would damage them, so 3.3 v operation is essential. I have a good stock of ATmega328P chips left over from the days when I was running Shrimping workshops. The ATmega328P works well at 3.3 volts, so long as the clock frequency is no more than 8 MHz . Most Arduinos run at 5v with a clock speed of 16 MHz, so there's work to do. 8 MHz clock issues If I2C is to work reliably at 8 MHz the Arduino needs to be configured for an 8 MHz clock, and it needs a bootloader that works at that clock rate. My first step was to wire up a working clone on a breadboard with an 8 MHz crystal and i