Use websockets to build a browser-based Digital Voltmeter
This is the third and final part of a series about building a browser-based six-channel Digital Voltmeter (DVM) using an Arduino and a Raspberry Pi.
Part one covered the software on the Arduino and the USB connection to the Raspberry Pi.
Part two showed you how to use the pyserial package to read that data into a Python program and print the results as they arrived.
In this final part you'll see how to use Joe Walnes' brilliantly simple websocketd. You'll see how you can turn the Python program into a server without writing any additional code! Finally, you'll view a web-page that displays the voltages in more or less real-time.
HTTP makes it easy for a web browser to ask for data from a web server. That approach is known as client pull because the client (the web browser) pulls (requests) the data from the server.
For the DVM application you want to use server push; in other words, you want to send changes in the voltages from a server on the Raspberry Pi to your browser. Websockets give you a simple, well-supported way of implementing web push.
You'll use websocketd to send the data from the Pi to the browser. websocketd is a really useful program which allows you to wrap any program or script that prints output and send that data to a browser via a websocket. (You can send data back from the browser as well, but the Web DVM doesn't need to do that.)
I followed four simple steps to install and use websocketd with the Python program form part 2.
As you'll see below, you just need to download and unzip a file and then run the command that starts the server.
If you're a confident GitHub user you can fork or clone the repository instead of downloading the zip file.
The code on GitHub assumes that you are using a Raspberry Pi with the hostname of raspberrypi. If you want to use your own choice of hostname, you'll need to make one simple change to the web page. I'll cover that in the final step of this post.
Open a terminal window on the Pi and move into a directory of your choice.
Type
You should see websocketd displaying messages as it starts up.
If you open a browser on rasberrypi:8080 you should see a web page like this >>
For demo purposes, I've connected the analog inputs to various fixed and variable voltages. For example, A0 is ground, A2 is connected to a light-dependent resistor and A3 is connected to the Arduino's 5V rail.
The web page is a basic html page with a little JavaScript.
Here's index.html:
The work is done by a small bit of JavaScript. The code
That's the bit of code you'll need to change if you want to use a different hstname for the Pi.
Whenever the websocket receives a message, the
In this three-part series you've met several useful techniques, and built the WebDVM. That's a useful piece of Test Equipment!
You can use the approach to make lots of different measurements, and there are several ways in which you can add functionality.
In a future blog post I'll show you how to add scrolling graphics to your web-based DVM.
Before that I'll be exploring the babelboard, another simple tool which you can use to quickly prototype physical computing applications.
The babelboard can be used with a Raspberry Pi or a Jetson Nano. Later posts will show how to build versions for the Adafruit feather and the BBC micro:bit.
The babelboard is a simple piece of open source hardware which you can build in an hour using readily available components.
To make sure you don't miss the next series, follow @rareblog on Twitter!
Part one covered the software on the Arduino and the USB connection to the Raspberry Pi.
Part two showed you how to use the pyserial package to read that data into a Python program and print the results as they arrived.
In this final part you'll see how to use Joe Walnes' brilliantly simple websocketd. You'll see how you can turn the Python program into a server without writing any additional code! Finally, you'll view a web-page that displays the voltages in more or less real-time.
Web sockets with websocketd
HTTP makes it easy for a web browser to ask for data from a web server. That approach is known as client pull because the client (the web browser) pulls (requests) the data from the server.
For the DVM application you want to use server push; in other words, you want to send changes in the voltages from a server on the Raspberry Pi to your browser. Websockets give you a simple, well-supported way of implementing web push.
You'll use websocketd to send the data from the Pi to the browser. websocketd is a really useful program which allows you to wrap any program or script that prints output and send that data to a browser via a websocket. (You can send data back from the browser as well, but the Web DVM doesn't need to do that.)
Four steps to use websocketd
I followed four simple steps to install and use websocketd with the Python program form part 2.
- I installed the Python program which you saw in Part two.
- Next I copied the websocketd binary program onto your Raspberry Pi.
- I installed a web page with some simple javscript as well as some html.
- To wrap the program, I started websocketd and told it where to find the program it should run. For the web DVM I wanted webstocketd to act as a conventional web server. To do that, I provided an extra parameter telling websocketd where to find the web pages to serve.
As you'll see below, you just need to download and unzip a file and then run the command that starts the server.
If you're a confident GitHub user you can fork or clone the repository instead of downloading the zip file.
The code on GitHub assumes that you are using a Raspberry Pi with the hostname of raspberrypi. If you want to use your own choice of hostname, you'll need to make one simple change to the web page. I'll cover that in the final step of this post.
Installing the Web DVM project on the Pi
Open a terminal window on the Pi and move into a directory of your choice.
Type
wget https://github.com/romilly/WebDVM/archive/master.zip
unzip master.zip
cd cd WebDVM-master/
./websocketd --port=8080 --staticdir=. ./read-dvm.py
You should see websocketd displaying messages as it starts up.
Testing the application
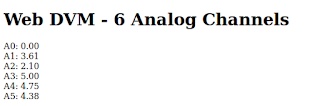
For demo purposes, I've connected the analog inputs to various fixed and variable voltages. For example, A0 is ground, A2 is connected to a light-dependent resistor and A3 is connected to the Arduino's 5V rail.
How does the web page work?
The web page is a basic html page with a little JavaScript.
Here's index.html:
<html lang="en"> <head> <meta charset="UTF-8"> <title>Web DVM</title> </head> <body> <h1>Web DVM - 6 Analog Channels</h1> <div>A0: <span id="A0"></span></div> <div>A1: <span id="A1"></span></div> <div>A2: <span id="A2"></span></div> <div>A3: <span id="A3"></span></div> <div>A4: <span id="A4"></span></div> <div>A5: <span id="A5"></span></div> <script> // setup websocket with callbacks var ws = new WebSocket('ws://raspberrypi:8080/'); ws.onopen = function() { }; ws.onclose = function() { }; ws.onmessage = function(event) { data = event.data.split('='); if (data[0].length === 2) { document.getElementById(data[0]).textContent = data[1]; } }; </script> </body> </html>
The work is done by a small bit of JavaScript. The code
var ws = new WebSocket('ws://raspberrypi:8080/');opens a websocket.
That's the bit of code you'll need to change if you want to use a different hstname for the Pi.
Whenever the websocket receives a message, the
ws.onmessagecode processes it and updates the contents of the web page.
Summary
In this three-part series you've met several useful techniques, and built the WebDVM. That's a useful piece of Test Equipment!
You can use the approach to make lots of different measurements, and there are several ways in which you can add functionality.
In a future blog post I'll show you how to add scrolling graphics to your web-based DVM.
The babelboard is coming
Before that I'll be exploring the babelboard, another simple tool which you can use to quickly prototype physical computing applications.
The babelboard can be used with a Raspberry Pi or a Jetson Nano. Later posts will show how to build versions for the Adafruit feather and the BBC micro:bit.
The babelboard is a simple piece of open source hardware which you can build in an hour using readily available components.
To make sure you don't miss the next series, follow @rareblog on Twitter!
Comments
Post a Comment